
folgendes Programm:
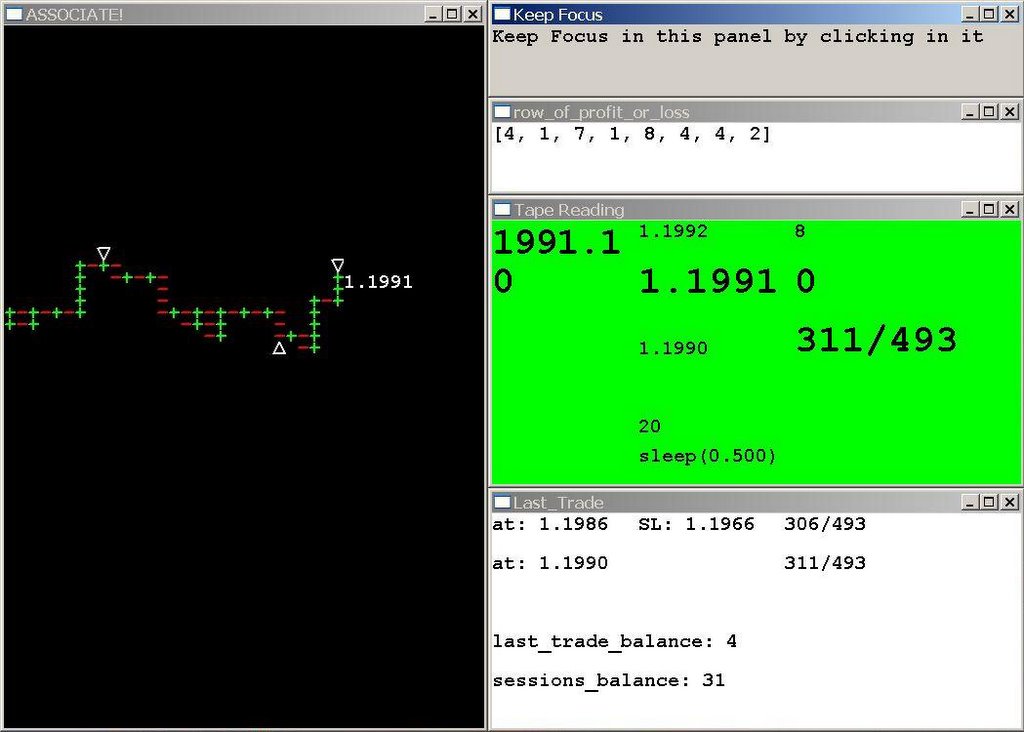
http://qweet.piranho.com/pictures.htm
Code: Alles auswählen
import wx
import time
import threading
import randomclient
from pprint import pprint
class row_of_profit_or_loss:
def __init__(self):
#INPUT
self.last_trade_balance = 0
#OUTPUT
self.row_length = 0
self.row = [0]
def sort_row_by_profit_or_loss(self):
self.row.insert(0, self.last_trade_balance)
if (self.row[0] > 0) & (self.row[1] <= 0):
self.row = [self.row[0]]
elif (self.row[0] < 0) & (self.row[1] >= 0):
self.row = [self.row[0]]
elif (self.row[0] == 0):
self.row = [self.row[0]]
def tell_row_length(self):
self.row_length = 0
for i in range(len(self.row)):
if self.row[0] > 0:
self.row_length += 1
elif self.row[0] < 0:
self.row_length -= 1
elif self.row[0] == 0:
self.row_length = 0
def do_action(self):
self.sort_row_by_profit_or_loss()
self.tell_row_length()
row_p_o_l = row_of_profit_or_loss()
class get_random_numbers:
def __init__(self):
# INPUT
self.how_many_numbers = 0
# OUTPUT
self.raw_random_numbers = []
self.new_random_numbers = []
"""
# FOR TESTING
self.new_random_numbers = []
for i in range(100):
self.new_random_numbers.append('1')
for i in range(100):
self.new_random_numbers.append('0')
"""
"""
# FOR TESTING
self.new_random_numbers = [
1,
-1, -1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1,
1,
-1, -1, -1, -1, -1,
1
]
"""
def get_numbers_from_client(self):
self.raw_random_numbers = randomclient.truerand(self.how_many_numbers/8, 'b')
def make_raw_to_new_numbers(self):
for i in range(self.how_many_numbers/8):
for j in range(8):
self.new_random_numbers.append( self.raw_random_numbers[i][j] )
def make_string_to_integer(self):
for i in range(len(self.new_random_numbers)):
if self.new_random_numbers[i] == '0':
self.new_random_numbers[i] = '-1'
self.new_random_numbers[i] = int(self.new_random_numbers[i])
def do_action(self):
self.get_numbers_from_client()
self.make_raw_to_new_numbers()
self.make_string_to_integer()
g_r_n = get_random_numbers()
class command_line:
def __init__(self):
self.how_many = input("Number of random-numbers? (only multiples of 8! max.80000): ")
g_r_n.how_many_numbers = self.how_many
g_r_n.do_action()
print "You're trading EUR/USD. You goal is to maximize your profit"
print "USAGE"
print "Click on the 'Keep-Focus' window to let the program receive the keystrokes"
print "The following keys take action in the program:"
print "<up-arrow>: buy order"
print "<down-arrow>: sell order"
print "If you bought and now you press 'down' you close your position"
print "Speed-Keys:"
print "<t> and <g>: 1"
print "<z> and <h>: 0.1"
print "<u> and <j>: 0.01"
print "<i> and <k>: 0.001"
print "<Plus> on num-block: Increase your Stop Loss"
print "<Minus> on num-block: Decrease your Stop Loss"
print "<enter> to stop the process"
print "<back-shift> to stop the process (should be the one over <enter>)"
print "<Key-a>: if your Stop Loss gets hitted you need to press this key to continue"
print "TO START PRESS <back-shift> (over <enter>)"
print "Good luck!"
c_l = command_line()
class make_calculations:
def __init__(self, new_random_numbers, start_price):
self.new_random_numbers = new_random_numbers
self.start_price = start_price
self.price_list = [self.start_price]
self.trend_list = []
self.up_trend_length = 0
self.down_trend_length = 0
self.trend_direction = ''
def calculate_all_prices(self):
for i in range(len(self.new_random_numbers)):
self.price_list.append (self.price_list[i]+self.new_random_numbers[i])
def calculate_current_trend(self):
for i in range(len(self.new_random_numbers)):
if (self.trend_direction != 'DOWN'):
if (self.new_random_numbers[i] == 1):
self.up_trend_length += 1
self.trend_direction = 'UP'
elif (self.trend_direction == 'UP'):
self.trend_list.append(self.up_trend_length)
self.up_trend_length = 0
self.trend_direction = 'DOWN'
if (self.new_random_numbers[i] == -1):
self.down_trend_length -= 1
self.trend_direction = 'DOWN'
elif (self.trend_direction == 'DOWN'):
self.trend_list.append(self.down_trend_length)
self.down_trend_length = 0
self.trend_direction = 'UP'
elif (self.trend_direction != 'UP'):
if (self.new_random_numbers[i] == -1):
self.down_trend_length -= 1
self.trend_direction = 'DOWN'
elif (self.trend_direction == 'DOWN'):
self.trend_list.append(self.down_trend_length)
self.down_trend_length = 0
self.trend_direction = 'UP'
if (self.new_random_numbers[i] == 1):
self.up_trend_length += 1
self.trend_direction = 'UP'
elif (self.trend_direction == 'UP'):
self.trend_list.append(self.up_trend_length)
self.up_trend_length = 0
self.trend_direction = 'DOWN'
if self.trend_direction == 'UP':
self.trend_list.append(self.up_trend_length)
elif self.trend_direction == 'DOWN':
self.trend_list.append(self.down_trend_length)
def do_action(self):
self.calculate_all_prices()
self.calculate_current_trend()
m_c = make_calculations(g_r_n.new_random_numbers, 2000)
m_c.do_action()
class Output_Panel_frame1(wx.Panel):
def __init__(self, parent):
wx.Panel.__init__(self, parent, -1, style=0)
self.SetBackgroundColour("WHITE")
x0 = 22
y0 = 40
self.one = wx.StaticText(self, -1, 'x')
self.one.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.one.MoveXY(0*x0, 0*y0)
self.ten = wx.StaticText(self, -1, 'x')
self.ten.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.ten.MoveXY(1*x0, 0*y0)
self.hundred = wx.StaticText(self, -1, 'x')
self.hundred.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.hundred.MoveXY(2*x0, 0*y0)
self.thousand = wx.StaticText(self, -1, 'x')
self.thousand.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.thousand.MoveXY(3*x0, 0*y0)
self.the_dot = wx.StaticText(self, -1, '.')
self.the_dot.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.the_dot.MoveXY(4*x0, 0*y0)
self.after_dot = wx.StaticText(self, -1, '1')
self.after_dot.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.after_dot.MoveXY(5*x0, 0*y0)
self.balance_left_edge = wx.StaticText(self, -1, 'B')
self.balance_left_edge.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.balance_left_edge.MoveXY(0*x0, 1*y0)
x1 = 150
y1 = 40
self.current_buy_price = wx.StaticText(self, -1, 'x.xxxx')
self.current_buy_price.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.current_buy_price.MoveXY(x1, 0*y1)
self.row_length = wx.StaticText(self, -1, 'row: x/x')
self.row_length.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.row_length.MoveXY(x1+160, 0*y1)
self.current_price = wx.StaticText(self, -1, 'x.xxxx')
self.current_price.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.current_price.MoveXY(x1, 1*y1)
self.current_sell_price = wx.StaticText(self, -1, 'x.xxxx')
self.current_sell_price.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.current_sell_price.MoveXY(x1, 3*y1)
self.balance = wx.StaticText(self, -1, 'B')
self.balance.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
self.balance.MoveXY(x1+160, 1*y1)
self.current_moment = wx.StaticText(self, -1, 'x/'+str(g_r_n.how_many_numbers))
self.current_moment.MoveXY(x1+160, 2*y1+20)
self.current_moment.SetFont(wx.Font(30, wx.MODERN, wx.NORMAL, wx.BOLD))
x2 = 150
y2 = 200
self.current_Stop_Loss_Difference = wx.StaticText(self, -1, '<current_Stop_Loss_Difference>')
self.current_Stop_Loss_Difference.MoveXY(x2, y2)
self.current_Stop_Loss_Difference.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.current_speed = wx.StaticText(self, -1, '<current_speed>')
self.current_speed.MoveXY(x2, y2+30)
self.current_speed.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
class Output_Panel_frame2(wx.Panel):
def __init__(self, parent):
wx.Panel.__init__(self, parent, -1, style=0)
self.SetBackgroundColour("WHITE")
x1 = 0
y1 = 40
self.long = wx.StaticText(self, -1, 'long at:')
self.long.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.long.MoveXY(x1, 0*y1)
self.upper_Stop_Loss = wx.StaticText(self, -1, 'SL: ')
self.upper_Stop_Loss.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.upper_Stop_Loss.MoveXY(x1+150, 0*y1)
self.current_moment_long = wx.StaticText(self, -1, 'x/'+str(g_r_n.how_many_numbers))
self.current_moment_long.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.current_moment_long.MoveXY(x1+300, 0*y1)
self.short = wx.StaticText(self, -1, 'short at:')
self.short.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.short.MoveXY(x1, 1*y1)
self.lower_Stop_Loss = wx.StaticText(self, -1, 'SL: ')
self.lower_Stop_Loss.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.lower_Stop_Loss.MoveXY(x1+150, 1*y1)
self.current_moment_short = wx.StaticText(self, -1, 'x/'+str(g_r_n.how_many_numbers))
self.current_moment_short.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.current_moment_short.MoveXY(x1+300, 1*y1)
self.last_trade_balance = wx.StaticText(self, -1, 'last_trade_balance: ')
self.last_trade_balance.MoveXY(x1, 3*y1)
self.last_trade_balance.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.sessions_balance = wx.StaticText(self, -1, 'sessions_balance: ')
self.sessions_balance.MoveXY(x1, 4*y1)
self.sessions_balance.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
class Input_Panel_frame3(wx.Panel):
def __init__(self, parent3):
wx.Panel.__init__(self, parent3, -1, style=0)
self.Bind(wx.EVT_CHAR, self.LogKeyEvent)
self.SetFocus()
self.text = wx.StaticText(self, -1, 'Keep Focus in this panel by clicking in it')
self.text.MoveXY(0, 0)
self.text.SetFont(wx.Font(15, wx.MODERN, wx.NORMAL, wx.BOLD))
self.Key_Enter = 13
self.Key_back = 8
self.Key_up = 317
self.Key_down = 319
self.Key_right = 318
self.Key_minus = 45
self.Key_plus = 43
self.Key_a = 97
self.Key_t = 116
self.Key_g = 103
self.Key_z = 122
self.Key_h = 104
self.Key_u = 117
self.Key_j = 106
self.Key_i = 105
self.Key_k = 107
self.PressedKeyCode = 0
self.Stop = 'YES'
self.buy_or_sell = ''
self.Stop_Loss_Difference = 20
self.visible_speed = ['2', '.', '0', '0', '0']
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[1] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
def reset_all(self):
self.PressedKeyCode = 0
self.buy_or_sell = ''
def do_action(self):
if self.PressedKeyCode == self.Key_Enter:
self.Stop = 'YES'
elif self.PressedKeyCode == self.Key_back:
self.Stop = 'NO'
elif self.PressedKeyCode == self.Key_plus:
self.Stop_Loss_Difference += 1
elif (self.PressedKeyCode == self.Key_minus) & (self.Stop_Loss_Difference > 3):
self.Stop_Loss_Difference -= 1
elif self.PressedKeyCode == self.Key_a:
c.Stop_Loss_reached = 'FALSE'
c.Core_stop = 'NO'
# T AND G
elif (self.PressedKeyCode == self.Key_t):
self.visible_speed[0] = str ( int(self.visible_speed[0]) + 1 )
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[1] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
c.Core_speed = float(self.visible_speed_as_string)
elif (self.PressedKeyCode == self.Key_g) & ( int(self.visible_speed[0]) > 0 ):
self.visible_speed[0] = str ( int(self.visible_speed[0]) - 1 )
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[1] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
c.Core_speed = float(self.visible_speed_as_string)
# Z AND H
elif (self.PressedKeyCode == self.Key_z) & ( int(self.visible_speed[2]) < 9 ):
self.visible_speed[2] = str ( int(self.visible_speed[2]) + 1 )
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[1] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
c.Core_speed = float(self.visible_speed_as_string)
elif (self.PressedKeyCode == self.Key_h) & ( int(self.visible_speed[2]) > 0 ):
self.visible_speed[2] = str ( int(self.visible_speed[2]) - 1 )
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[1] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
c.Core_speed = float(self.visible_speed_as_string)
# U AND J
elif (self.PressedKeyCode == self.Key_u) & ( int(self.visible_speed[3]) < 9 ):
self.visible_speed[3] = str ( int(self.visible_speed[3]) + 1 )
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[1] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
c.Core_speed = float(self.visible_speed_as_string)
elif (self.PressedKeyCode == self.Key_j) & ( int(self.visible_speed[3]) > 0 ):
self.visible_speed[3] = str ( int(self.visible_speed[3]) - 1 )
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[1] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
c.Core_speed = float(self.visible_speed_as_string)
# I AND K
elif (self.PressedKeyCode == self.Key_i) & ( int(self.visible_speed[4]) < 9 ):
self.visible_speed[4] = str ( int(self.visible_speed[4]) + 1 )
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[4] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
c.Core_speed = float(self.visible_speed_as_string)
elif (self.PressedKeyCode == self.Key_k) & ( int(self.visible_speed[4]) > 0 ):
self.visible_speed[4] = str ( int(self.visible_speed[4]) - 1 )
self.visible_speed_as_string = (self.visible_speed[0] + self.visible_speed[1] + self.visible_speed[2] +
self.visible_speed[3] + self.visible_speed[4])
c.Core_speed = float(self.visible_speed_as_string)
O_P_f1.current_Stop_Loss_Difference.SetLabel(str(self.Stop_Loss_Difference))
O_P_f1.current_speed.SetLabel('sleep('+self.visible_speed_as_string+')')
if c.Stop_Loss_reached == 'FALSE':
if self.PressedKeyCode == self.Key_up:
self.buy_or_sell = 'BUY'
elif self.PressedKeyCode == self.Key_down:
self.buy_or_sell = 'SELL'
if not workerthread.isAlive():
global workerthread
workerthread = threading.Thread(target=c.run)
workerthread.start()
def LogKeyEvent(self, evt):
self.PressedKeyCode = evt.GetKeyCode()
self.do_action()
class Core:
def __init__(self):
self.Core_stop = 'NO'
self.Core_speed = 2.000
self.current_moment = 0
self.current_buy_price = 2001
self.current_price = 2000
self.current_sell_price = 1999
self.string_current_price = str(self.current_price)
self.bought_or_sold = ''
self.remember_price = 0
self.Stop_Loss_absolute = 0
self.current_balance = 0
self.last_trade_balance = 0
self.sessions_balance = 0
self.Stop_Loss_reached = 'FALSE'
self.row_of_profit_loss = []
self.row_length = 0
self.only_profit_only_loss = ''
def run(self):
while (I_P_f3.Stop == 'NO') & (self.Core_stop == 'NO'):
if self.Core_stop == 'NO':
O_P_f1.SetBackgroundColour("WHITE")
O_P_f1.Refresh()
self.current_price += m_c.trend_list[self.current_moment]
self.current_buy_price = self.current_price + 1
self.current_sell_price = self.current_price - 1
self.string_current_price = str(self.current_price)
if (I_P_f3.buy_or_sell == 'BUY') & (self.bought_or_sold == ''):
O_P_f2.short.SetLabel('')
O_P_f2.long.SetLabel('')
O_P_f2.upper_Stop_Loss.SetLabel('')
O_P_f2.lower_Stop_Loss.SetLabel('')
O_P_f2.current_moment_long.SetLabel('')
O_P_f2.current_moment_short.SetLabel('')
self.remember_price = self.current_buy_price
self.bought_or_sold = 'BOUGHT'
self.Stop_Loss_absolute = self.remember_price - I_P_f3.Stop_Loss_Difference
self.Core_stop = 'YES'
O_P_f2.long.SetLabel('at: '+'1.'+str(self.remember_price))
O_P_f2.upper_Stop_Loss.SetLabel('SL: '+'1.'+str(self.Stop_Loss_absolute))
O_P_f2.current_moment_long.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
elif (I_P_f3.buy_or_sell == 'SELL') & (self.bought_or_sold == ''):
O_P_f2.short.SetLabel('')
O_P_f2.long.SetLabel('')
O_P_f2.upper_Stop_Loss.SetLabel('')
O_P_f2.lower_Stop_Loss.SetLabel('')
O_P_f2.current_moment_short.SetLabel('')
O_P_f2.current_moment_long.SetLabel('')
self.remember_price = self.current_sell_price
self.bought_or_sold = 'SOLD'
self.Stop_Loss_absolute = self.remember_price + I_P_f3.Stop_Loss_Difference
self.Core_stop = 'YES'
O_P_f2.short.SetLabel('at: '+'1.'+str(self.remember_price))
O_P_f2.lower_Stop_Loss.SetLabel('SL: '+'1.'+str(self.Stop_Loss_absolute))
O_P_f2.current_moment_short.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
if self.bought_or_sold == 'BOUGHT':
self.current_balance = self.current_sell_price - self.remember_price
elif self.bought_or_sold == 'SOLD':
self.current_balance = self.remember_price - self.current_buy_price
if (I_P_f3.buy_or_sell == 'SELL') & (self.bought_or_sold == 'BOUGHT'):
self.bought_or_sold = ''
self.last_trade_balance = self.current_balance
self.sessions_balance += self.last_trade_balance
print self.current_balance
if self.current_balance > 0:
O_P_f1.SetBackgroundColour("GREEN")
O_P_f1.Refresh()
elif self.current_balance < 0:
O_P_f1.SetBackgroundColour("RED")
O_P_f1.Refresh()
self.current_balance = 0
self.Core_stop = 'YES'
row_p_o_l.last_trade_balance = self.last_trade_balance
row_p_o_l.do_action()
self.row_length = row_p_o_l.row_length
O_P_f2.short.SetLabel('at: '+'1.'+str(self.current_sell_price))
O_P_f2.last_trade_balance.SetLabel('last_trade_balance: '+str(self.last_trade_balance))
O_P_f2.sessions_balance.SetLabel('sessions_balance: '+str(self.sessions_balance))
O_P_f2.current_moment_short.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
elif (I_P_f3.buy_or_sell == 'BUY') & (self.bought_or_sold == 'SOLD'):
self.bought_or_sold = ''
self.last_trade_balance = self.current_balance
self.sessions_balance += self.last_trade_balance
print self.current_balance
if self.current_balance > 0:
O_P_f1.SetBackgroundColour("GREEN")
O_P_f1.Refresh()
elif self.current_balance < 0:
O_P_f1.SetBackgroundColour("RED")
O_P_f1.Refresh()
self.current_balance = 0
self.Core_stop = 'YES'
row_p_o_l.last_trade_balance = self.last_trade_balance
row_p_o_l.do_action()
self.row_length = row_p_o_l.row_length
O_P_f2.long.SetLabel('at: '+'1.'+str(self.current_buy_price))
O_P_f2.last_trade_balance.SetLabel('last_trade_balance: '+str(self.last_trade_balance))
O_P_f2.sessions_balance.SetLabel('sessions_balance: '+str(self.sessions_balance))
O_P_f2.current_moment_long.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
if (self.bought_or_sold == 'BOUGHT'):
if self.current_sell_price <= self.Stop_Loss_absolute:
self.bought_or_sold = ''
self.Stop_Loss_reached = 'TRUE'
self.last_trade_balance = self.Stop_Loss_absolute - self.remember_price
self.sessions_balance += self.last_trade_balance
self.current_balance = 0
row_p_o_l.last_trade_balance = self.last_trade_balance
row_p_o_l.do_action()
self.row_length = row_p_o_l.row_length
O_P_f1.SetBackgroundColour("RED")
O_P_f1.Refresh()
O_P_f2.short.SetLabel('at: '+'1.'+str(self.Stop_Loss_absolute))
O_P_f2.last_trade_balance.SetLabel('last_trade_balance: '+str(self.last_trade_balance))
O_P_f2.sessions_balance.SetLabel('sessions_balance: '+str(self.sessions_balance))
O_P_f2.current_moment_short.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
elif (self.bought_or_sold == 'SOLD'):
if self.current_buy_price >= self.Stop_Loss_absolute:
self.bought_or_sold = ''
self.Stop_Loss_reached = 'TRUE'
self.last_trade_balance = self.remember_price - self.Stop_Loss_absolute
self.sessions_balance += self.last_trade_balance
self.current_balance = 0
row_p_o_l.last_trade_balance = self.last_trade_balance
row_p_o_l.do_action()
self.row_length = row_p_o_l.row_length
O_P_f1.SetBackgroundColour("RED")
O_P_f1.Refresh()
O_P_f2.long.SetLabel('at: '+'1.'+str(self.Stop_Loss_absolute))
O_P_f2.last_trade_balance.SetLabel('last_trade_balance: '+str(self.last_trade_balance))
O_P_f2.sessions_balance.SetLabel('sessions_balance: '+str(self.sessions_balance))
O_P_f2.current_moment_long.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
O_P_f1.row_length.SetLabel (str(self.row_length))
O_P_f1.balance_left_edge.SetLabel (str(self.current_balance))
O_P_f1.balance.SetLabel (str(self.current_balance))
O_P_f1.one.SetLabel (self.string_current_price[3])
O_P_f1.ten.SetLabel (self.string_current_price[2])
O_P_f1.hundred.SetLabel (self.string_current_price[1])
O_P_f1.thousand.SetLabel (self.string_current_price[0])
O_P_f1.current_buy_price.SetLabel('1.'+str(self.current_buy_price))
O_P_f1.current_price.SetLabel('1.'+str(self.current_price))
O_P_f1.current_sell_price.SetLabel('1.'+str(self.current_sell_price))
O_P_f1.current_moment.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
O_P_f4.row.SetLabel(str(row_p_o_l.row))
O_P_f5.current_price = self.current_price
O_P_f5.current_trend = m_c.trend_list[self.current_moment]
O_P_f5.do_action()
print I_P_f3.buy_or_sell
I_P_f3.reset_all()
if self.Stop_Loss_reached == 'TRUE':
self.Core_stop = 'YES'
self.current_moment += 1
time.sleep( self.Core_speed )
if self.current_moment == len(m_c.trend_list):
self.Core_stop = 'YES'
if self.bought_or_sold == 'BOUGHT':
self.bought_or_sold = ''
self.last_trade_balance = self.current_balance
self.sessions_balance += self.last_trade_balance
row_p_o_l.last_trade_balance = self.last_trade_balance
row_p_o_l.do_action()
self.row_length = row_p_o_l.row_length
O_P_f2.short.SetLabel('at: '+'1.'+str(self.current_sell_price))
O_P_f2.last_trade_balance.SetLabel('last_trade_balance: '+str(self.last_trade_balance))
O_P_f2.sessions_balance.SetLabel('sessions_balance: '+str(self.sessions_balance))
O_P_f2.current_moment_short.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
elif self.bought_or_sold == 'SOLD':
self.bought_or_sold = ''
self.last_trade_balance = self.current_balance
self.sessions_balance += self.last_trade_balance
row_p_o_l.last_trade_balance = self.last_trade_balance
row_p_o_l.do_action()
self.row_length = row_p_o_l.row_length
O_P_f2.long.SetLabel('at: '+'1.'+str(self.current_buy_price))
O_P_f2.last_trade_balance.SetLabel('last_trade_balance: '+str(self.last_trade_balance))
O_P_f2.sessions_balance.SetLabel('sessions_balance: '+str(self.sessions_balance))
O_P_f2.current_moment_long.SetLabel(str(self.current_moment)+'/'+str(len(m_c.trend_list)-1))
O_P_f1.row_length.SetLabel (str(self.row_length))
O_P_f4.row.SetLabel (str(row_p_o_l.row))